Bit Masking and Shift Operations
Instructions
Write a C program that reads 5 numbers from the user input in decimal format and packs its bits to a single 32 bit value that will be written to the standard output as a hexadecimal value. The program should handle both positive and negative numbers.
A function with the signature void pack(int input, int start_bit, int end_bit, int *val) might be a good start for this exercise.
The previously presented functions read and write can be used for reading/writing information from/to the standard input/output. The code snippet below can be used to write the resulting hexadecimal value to STDOUT (note that it uses the write function).
void hex_code(int val){
char hex[11];
unsigned int uval = (unsigned int) val, aux;
hex[0] = '0';
hex[1] = 'x';
hex[10] = '\n';
for (int i = 9; i > 1; i--){
aux = uval % 16;
if (aux >= 10)
hex[i] = aux - 10 + 'A';
else
hex[i] = aux + '0';
uval = uval / 16;
}
write(1, hex, 11);
}
Input
- 5 signed 4-digit decimal numbers separated by spaces (' '), followed by a newline character ('\n'). The whole input takes up 30 bytes.
- String Format - "SDDDD SDDDD SDDDD SDDDD SDDDD\n"
- S: sign, can be either '+' for positive numbers and '-' for negative.
- D: a decimal digit, (0-9)
Output
After reading all 5 numbers, you must pack their least significant bits (LSB) following the rules listed below:
- 1st number: 3 LSB => Bits 0 - 2
- 2nd number: 8 LSB => Bits 3 - 10
- 3rd number: 5 LSB => Bits 11 - 15
- 4th number: 5 LSB => Bits 16 - 20
- 5th number: 11 LSB => Bits 21 - 31
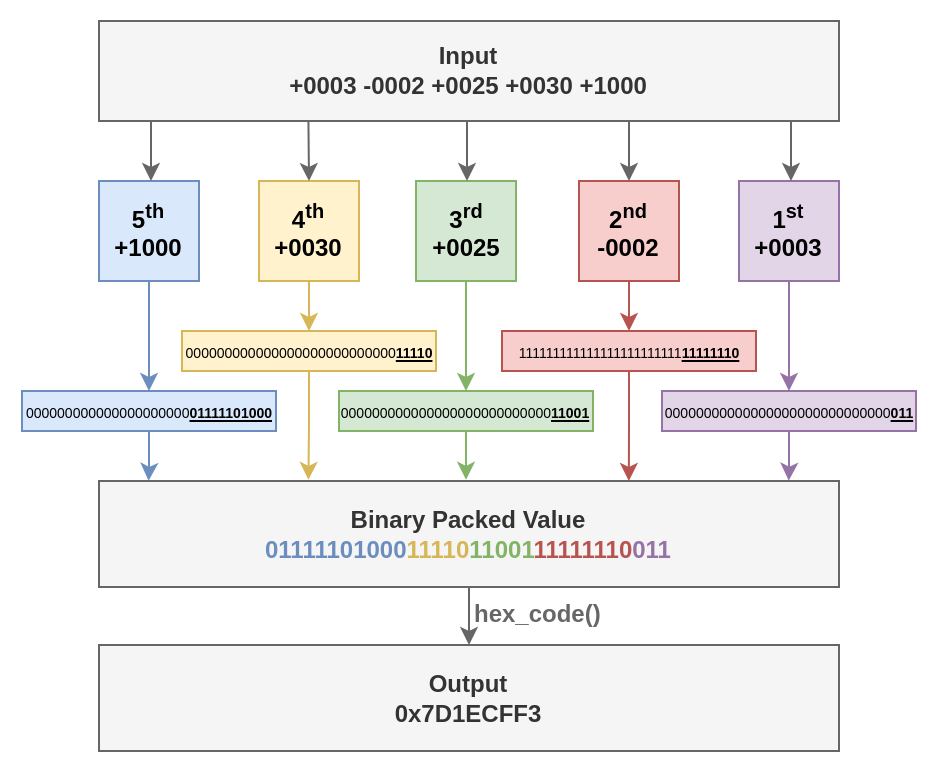
Figure 5.1.1: Bit extraction from 5 32-bit inputs to constitute a single 32-bit output
Examples
Test Case | Input | Output |
---|---|---|
1 | -0001 -0001 -0001 -0001 -0001 | 0xFFFFFFFF |
2 | +0001 +0001 -0001 -0001 -0001 | 0xFFFFF809 |
3 | +0003 -0002 +0025 +0030 +1000 | 0x7D1ECFF3 |
4 | +9999 +9999 +9999 +9999 +9999 | 0xE1EF787F |
Notes and Tips
- You may use the C operator for bit manipulation (<<, >>, |, &, ^, …) to implement your function. Suggested readings:
- You can test your code using the simulator's assistant from this link.